In this tutorial, we will learn how to send text messages from a Raspberry Pi. These text messages can contain useful information which we can use to trigger events elsewhere. We can also set it up in such a way that it sends alerts to notify us when sensors reach certain threshold values.
In this tutorial, we will connect a Raspberry Pi to IFTTT, so that we can send text messages when a PIR motion sensor connected to the Raspberry Pi detects motion.
What is IFTTT?
IFTTT stands for If This, Then That. As the name suggests, if a condition is met in our application, the service will perform some action. We can use IFTTT with the Raspberry Pi to perform a variety of tasks, including sending text messages.
How to Setup IFTTT
Follow the steps below to setup a Raspberry Pi for IFTTTGo to the IFTTT website to create an account:
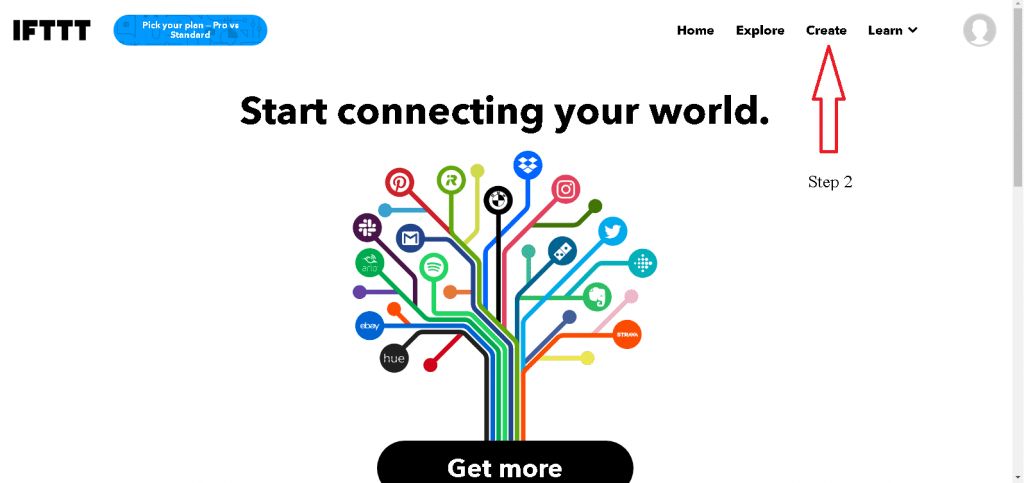
We can now create an action. Simply click on the “Create” tab as shown above. Then select the trigger for our IFTTT. Click on the “IF THIS” tab.
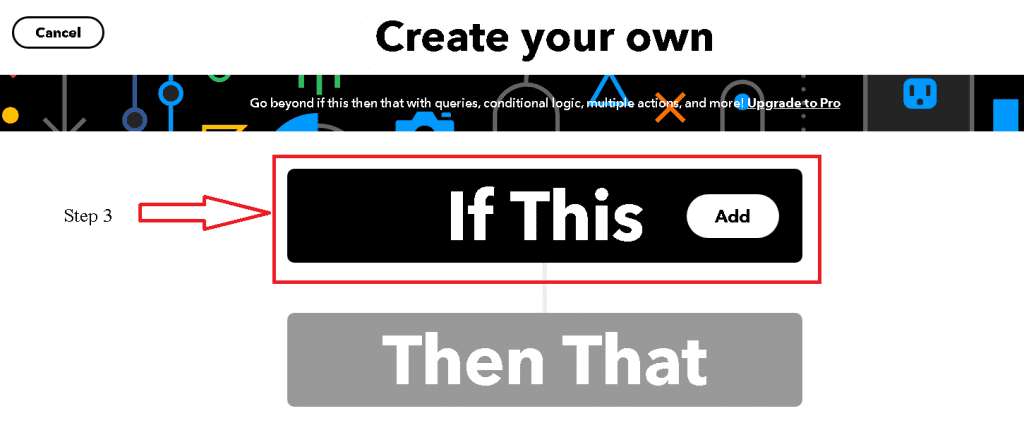
Now, choose a service for the trigger. For this demonstration, we will use the Webhook service.
IFTTT Webhooks
Webhooks allow web apps to communicate with each other. They are more like SMS notifications, but in this case, they are sent from one app to another when something happens. Just like regular SMS, webhooks transfer messages in real-time. Search for webhooks and click on the webhooks icon to select it as a service. Whenever a triggering event occurs on our Raspberry Pi, the webhook identifies the event and sends information to the specified URL in the form of an HTTP request.
Click on the “Receive a web request” trigger. It’s the only available trigger that you will find under Webhooks.
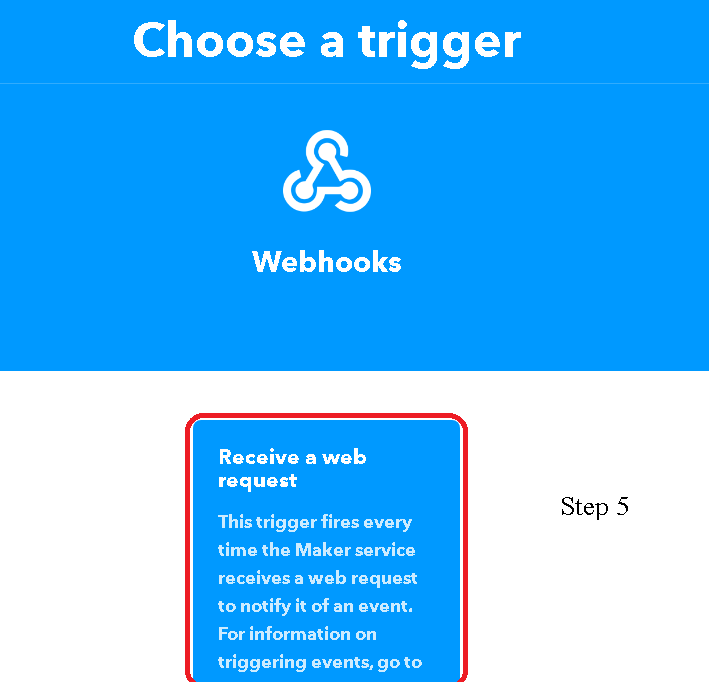
Click “Connect”, enter “Event name” and click “Create trigger”.
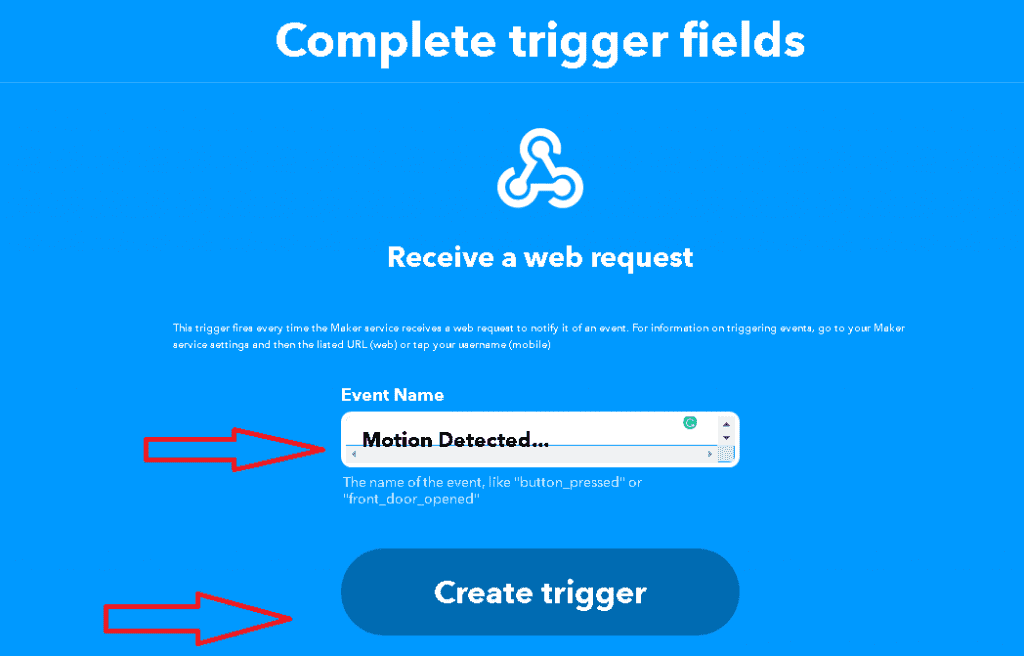
Now, we need to create an action when our “If This” happens. So click on “Then That” to select a service for our action.
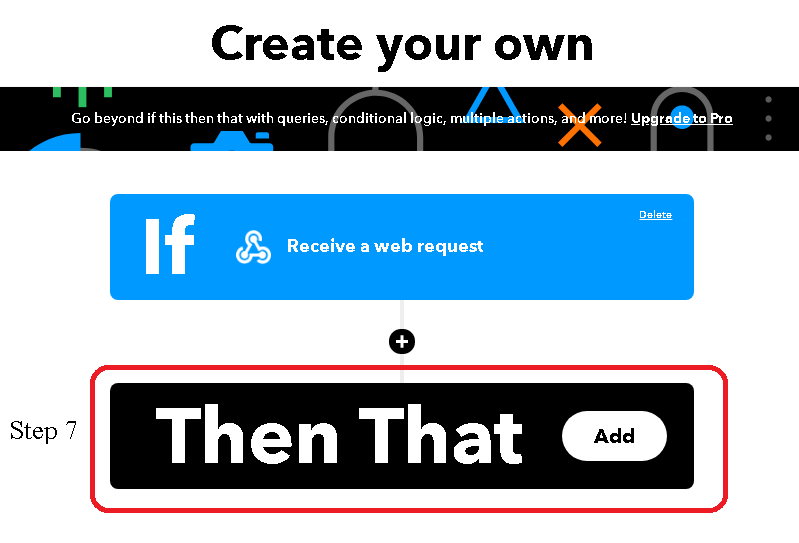
For this tutorial, we will use the “Notifications” service. Search for the notifications service and click on the icon. This will take you to a screen where you can select the type of notifications you wish to receive.
We intend to receive simple notifications, so we will select “Send a notification from the IFTTT app.”
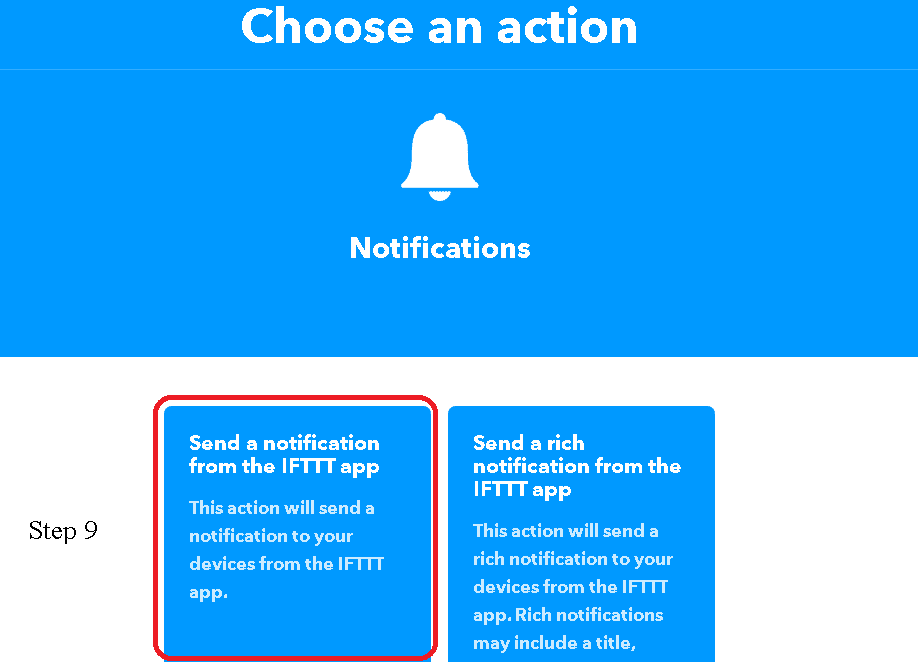
On the next page, click “Connect,” then enter the message you want to send when an event occurs. For example, “The Raspberry Pi has detected motion.” Do not worry about the default variables. For now, we just write the message that we want to send when the Raspberry Pi detects motion.
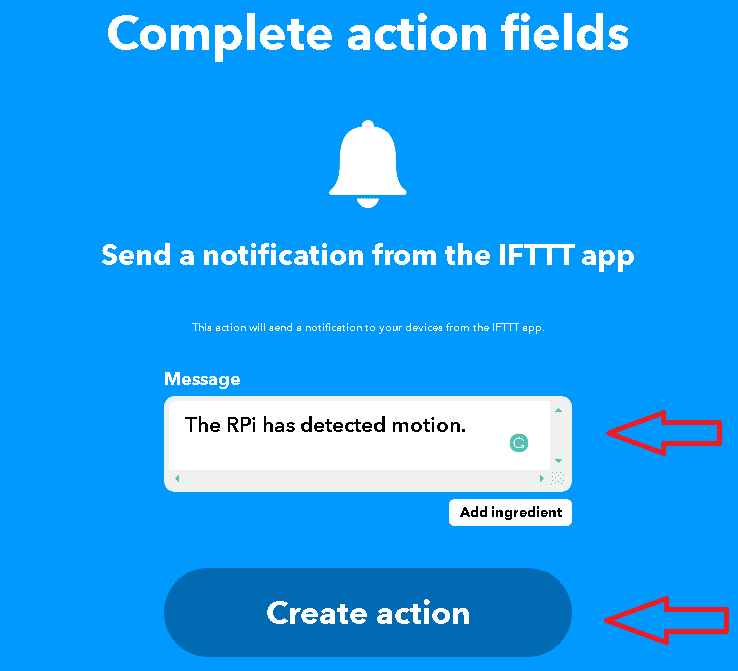
On the next page, click “Continue” then click the “Finish” button and enter your phone number when prompted.
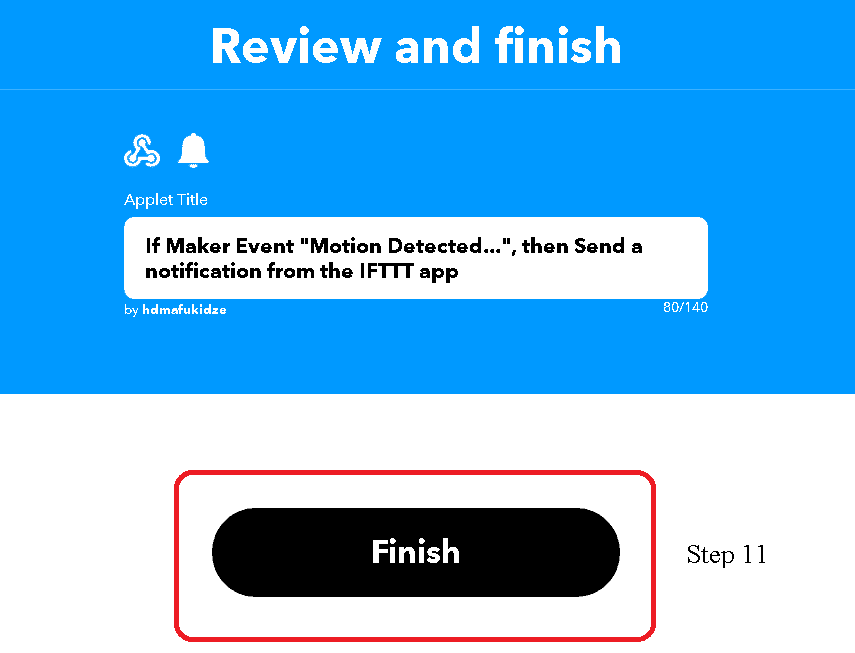
Click on the Webhooks icon to take you back to the Webhooks page.
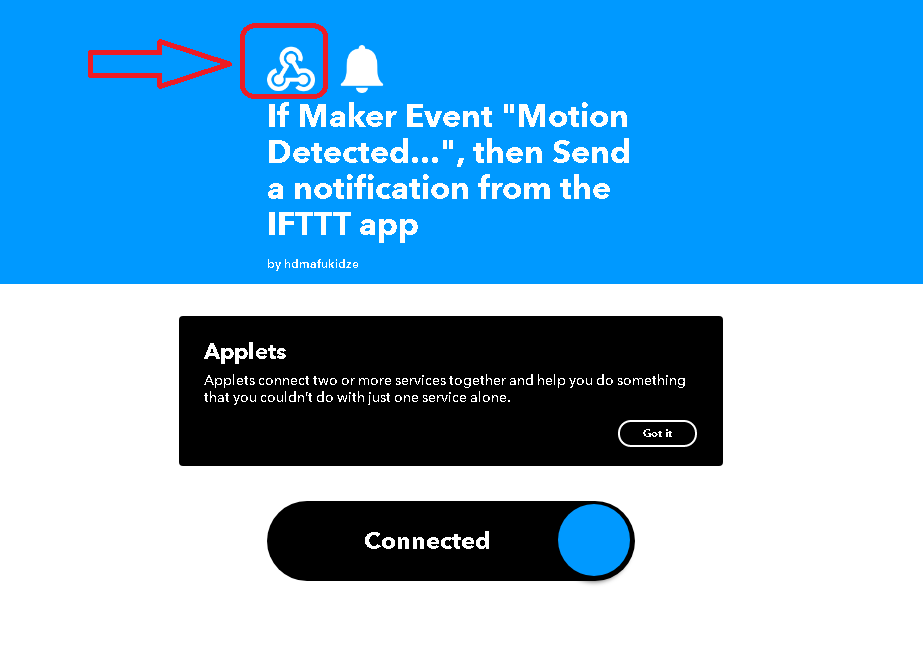
At the top right-hand corner of the screen, click “Documentation.”
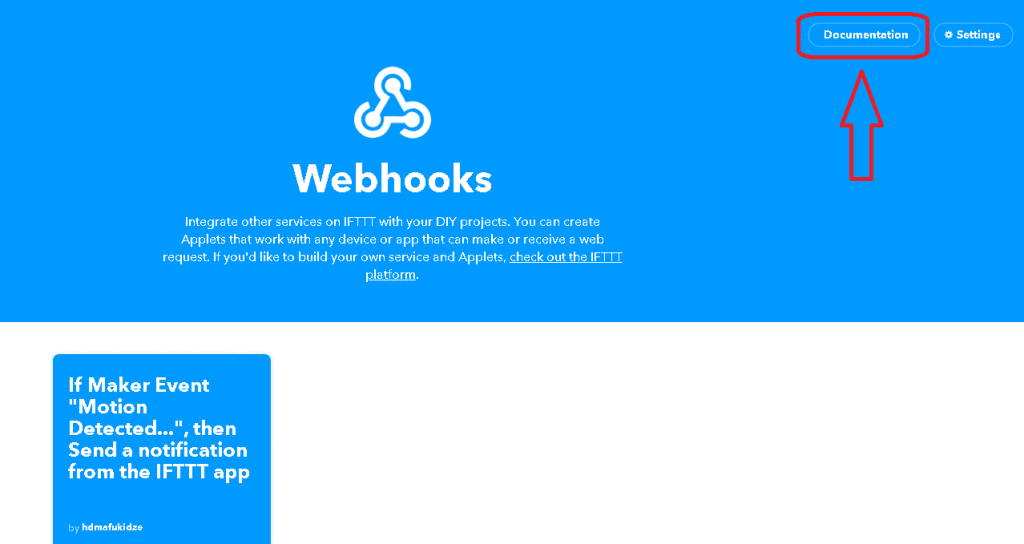
On the next page, copy your API key and the URL where we will send the webhooks notifications.
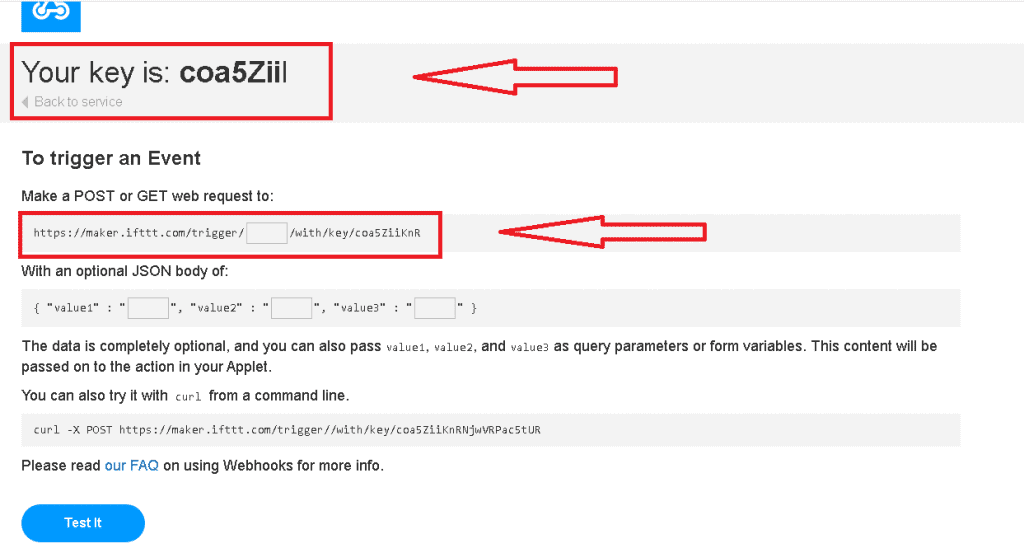
So far so good! The web service is set up and we are now ready to configure the Raspberry Pi to send the alerts.
How to Connect the Raspberry Pi to IFTTT
First we need to install the “requests” module. Type sudo pip3 install requests
and run on your command prompt. Then, create a new Python file with the command: sudo nano ifttt.py
.
Next, we need to create a POST request to the webhook URL. We need to know the event name that we used earlier on, and we need to have the API key ready. I have highlighted these details in the image above.
Add the following code to this file:
import requests
requests.post('https://maker.ifttt.com/trigger/{EventName} /with/key/{YourAPIKey}')
Save the file. But before you execute the script, install the IFTTT app.
To install the IFTTT app on your Raspberry Pi. The app is available on the App Store and Google Play store. After the app is installed, execute the script with this command:
sudo python3 ifttt.py
Almost immediately, you should receive a notification on your device:
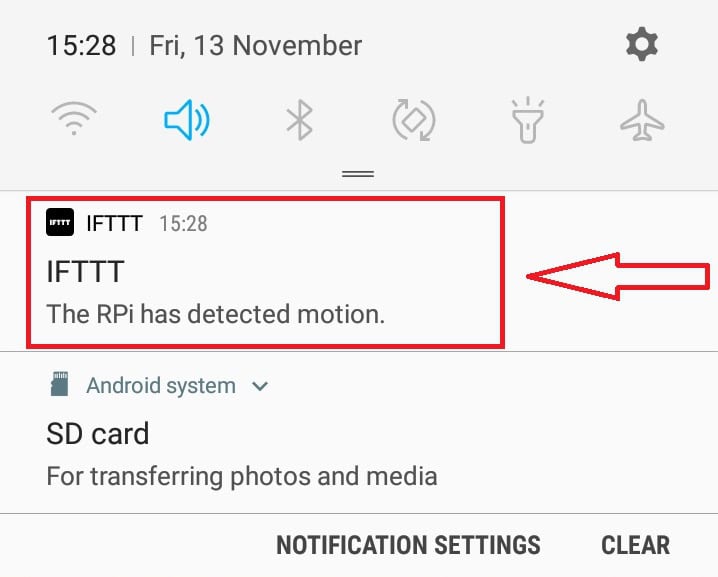
If not, check if the API key and the event name are correct. And, of course, make sure your Raspberry Pi is connected to the internet.
How Webhook Works
HTML GET Requests
The GET method is used to request data from a URL:
GET https://ifttt.com/connect/{connection_id}?
HTML POST Requests
We use the POST method to send data to a specific URL. For this example, we are sending the event name and the API key to the IFTTT URL.
This is the code that we use to make the POST request:
requests.post('https://maker.ifttt.com/trigger/{EventName} /with/key/{YourAPIKey}')
Send a Text Message When a PIR Sensor Detects Motion
In this project, we will send a text message when motion is detected by a PIR sensor connected to the Raspberry Pi. In a previous tutorial, we learned how to detect motion on the Raspberry Pi with a PIR sensor. In this article, we are going to edit that code to include the IFTTT requests.
These are the parts we will need:
Once you have the parts, connect the PIR sensor to the Raspberry Pi like this:
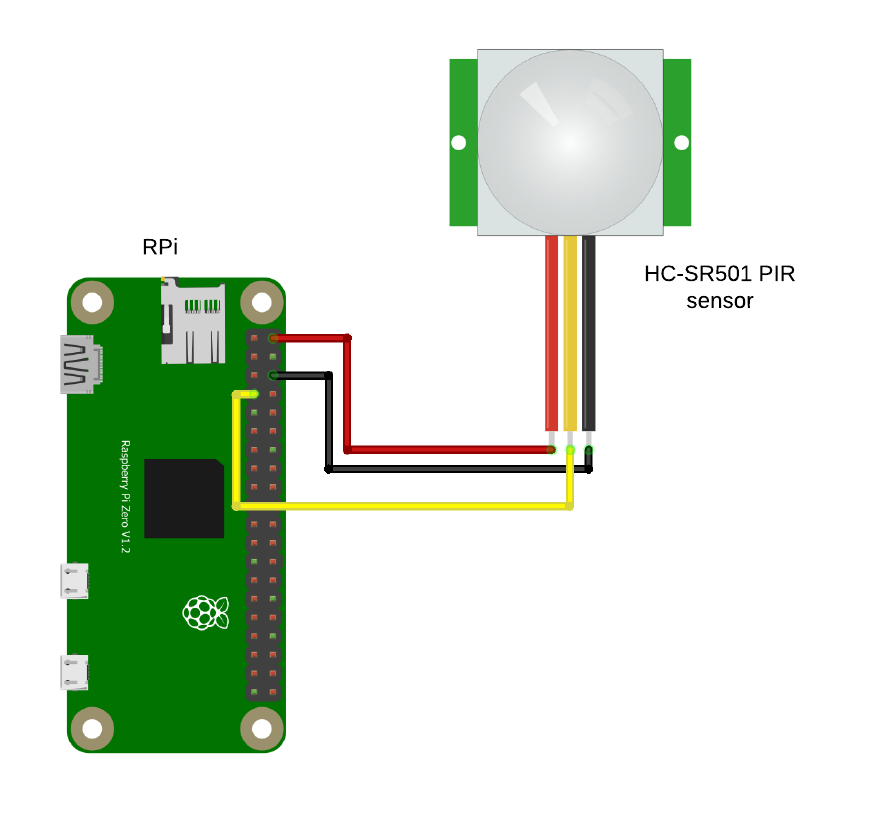
The Code
Save the following code to a file with a “.py” extension:
import RPi.GPIO as GPIO
import time
import requests
GPIO.setmode(GPIO.BCM)
pirPin = 4
GPIO.setup(PirPin, GPIO.IN)
time.sleep(2)
while True:
if GPIO.input(pirPin):
print("Motion Detected")
requests.post('https://maker.ifttt.com/trigger/MotionDetected/with/key/coa5ZiiKnNjwVRP')
time.sleep(1)
print("No one")
We use an if loop to monitor the PIR sensor’s output pin, which is connected to Raspberry Pi pin 4. When a signal has been detected, we send a POST request to the IFTTT URL with the event name and the API key:
requests.post('https://maker.ifttt.com/trigger/MotionDetected/with/key/coa5ZiiKnNjwVRP')
If you have setup everything correctly, you should get the following notifications on your device.
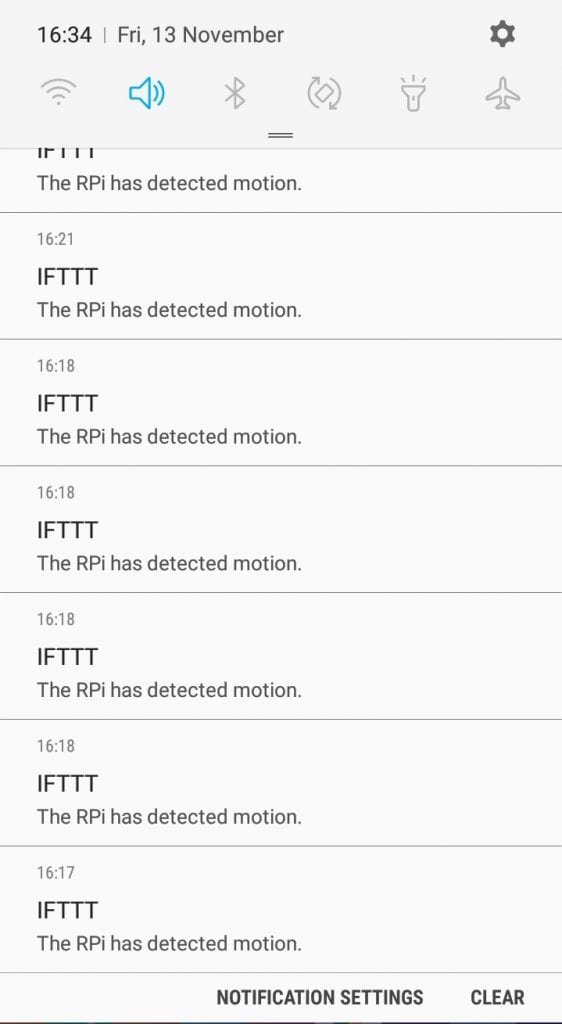
Hope this article has helped you to send text messages with your Raspberry Pi using IFTTT. Be sure to leave a comment below if you have questions about anything.