In this article, we will see how to use two different types of piezoelectric buzzers on the Arduino. Piezoelectric buzzers produce a loud noise. When connected to the Arduino they can be used as an alarm or notification when a motion sensor is triggered, or when a sensor reaches a certain value. They can also be programed to produce musical notes.
Watch the video for this tutorial here:
There are two types of piezoelectric buzzers that are commonly used in electronics projects – active buzzers and passive buzzers. Active buzzers are called active because they only need a DC voltage to produce sound. Passive buzzers need an AC voltage to produce sound.
Active buzzers and passive buzzers look very similar:
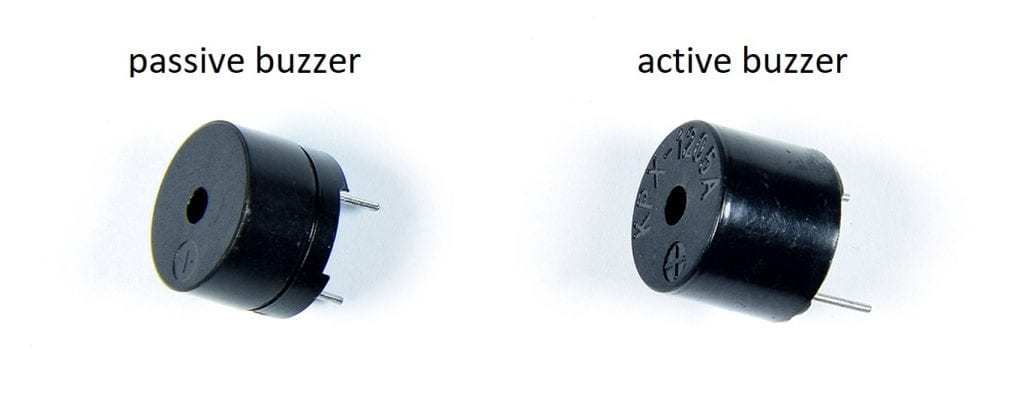
An easy way to tell active and passive buzzers apart is by connecting them to a DC voltage source like a 9 volt battery. The buzzers are polarized, so check which terminal is positive and which terminal is negative before connecting it to a battery.
When you connect a passive buzzer to a battery, the buzzer will make a sharp clicking sound. But when you connect an active buzzer to a battery, the buzzer will make a loud buzzing noise.
How Active Buzzers and Passive Buzzers Work
Active and passive buzzers are types of magnetic buzzers. Inside the buzzer, there is a coil of wire that’s connected to the buzzer’s pins. There is also a round magnet that surrounds the wire coil. A thin metal film with a metal weight attached to the top sits above the round magnet and wire coil. When pulses of current are applied to the wire coil, magnetic inductance causes the metal weight and metal film to vibrate up and down. The vibration of the metal film produces sound waves:
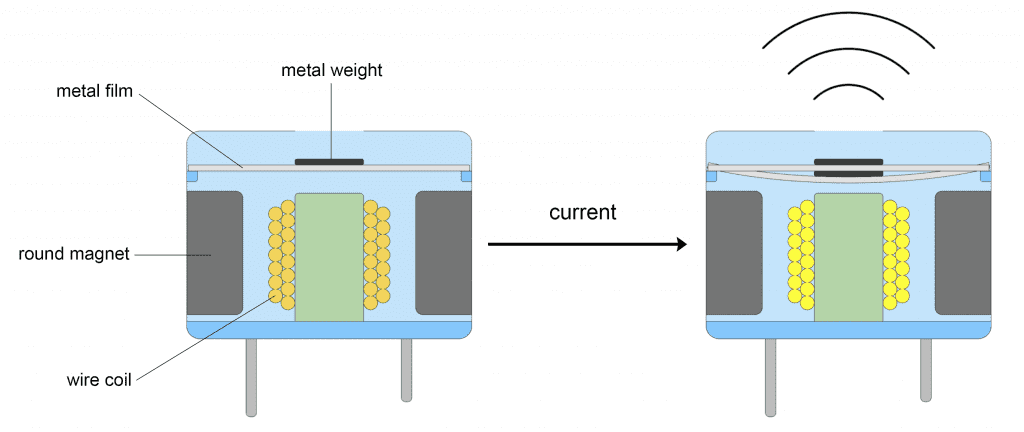
How to Connect an Active Buzzer to the Arduino
Let’s build an example project that will control an active buzzer with the press of a button.
Here are the parts you will need:
To connect the active buzzer and push button to the Arduino, follow the diagram below:
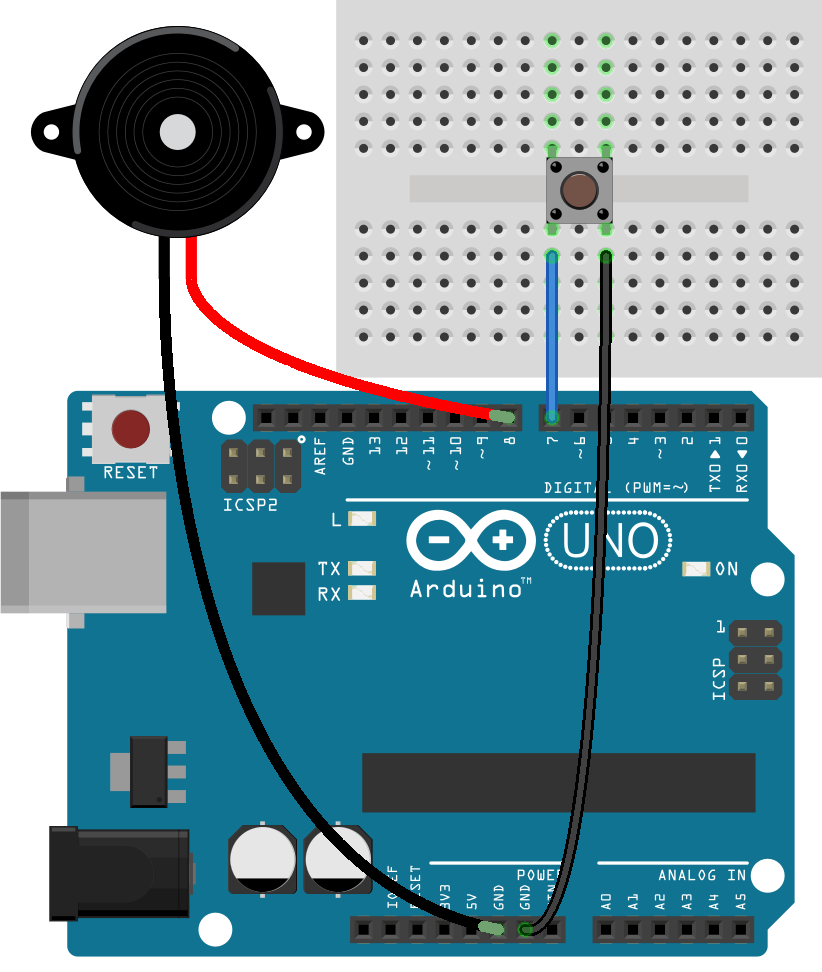
How to Program an Active Buzzer on the Arduino
Once your circuit is connected, upload this code to the Arduino:
int buzzerPin = 8;
int buttonPin = 7;
void setup() {
pinMode(buzzerPin, OUTPUT);
pinMode(buttonPin, INPUT_PULLUP);
}
void loop() {
int buttonState = digitalRead(buttonPin);
if (buttonState == LOW) {
digitalWrite(buzzerPin, HIGH);
}
if (buttonState == HIGH) {
digitalWrite(buzzerPin, LOW);
}
}
Explanation of the Code
Active buzzers are programmed just like LEDs. You digital write the buzzer pin HIGH to turn it on, and write the buzzer pin LOW to turn it off.
At the top of the sketch we declare two pin variables. The first pin variable is called buzzerPin
and is set equal to Arduino pin 8. The other pin variable is called buttonPin
and is set equal to pin 7.
In the setup()
section the buzzerPin
is set as an OUTPUT with the pinMode()
function. The buttonPin
is set as an INPUT with internal pullup resistor by using INPUT_PULLUP as the second argument in the pinMode()
function.
In the loop()
section we take a digital read of the buttonPin
and store the value in a local int variable called buttonState
. Then we have two if
statements that say “if the buttonState
is LOW then digital write the buzzer pin HIGH, and if the buttonState
is HIGH then digital write the buzzer pin LOW”.
After you connect the circuit and upload the code, you should see that pressing the button turns the buzzer on.
How to Use a Passive Buzzer on the Arduino
One advantage of passive buzzers over active buzzers is that you can control the tone or pitch of the sound produced by the buzzer. With active buzzers only one tone is possible, but with passive buzzers any tone within the dynamic range of the buzzer is possible.
Passive buzzers need a square wave signal to produce sound. By changing the frequency of the square wave you can change the pitch of the sound.
The Arduino has a built in function called tone()
that generates square waves at a range of frequencies:
tone(pin, frequency, duration);
The tone()
function takes three parameters – the pin number you want to send the square wave to, the frequency of the tone in hertz, and optionally the duration of the tone in milliseconds.
The noTone()
function can be used to turn off the tone()
function:
noTone();
How to Connect a Passive Buzzer to the Arduino
Let’s build a circuit that cycles through a set of musical notes from A to G.
You will need these parts:
First, connect a passive buzzer to the Arduino like this:
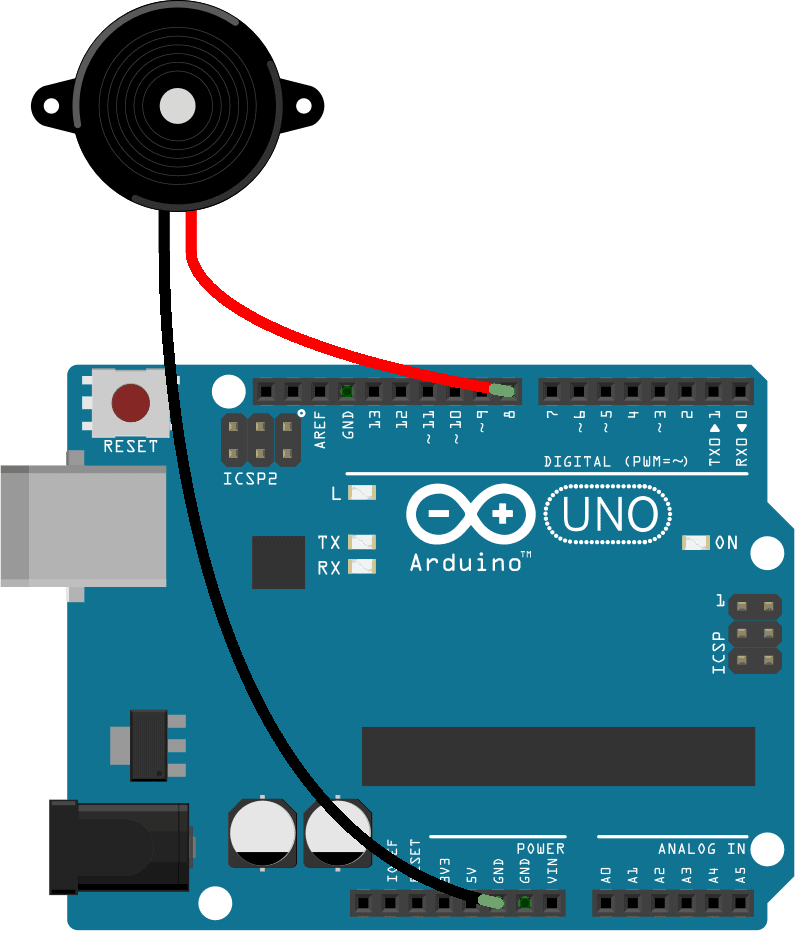
How to Program a Passive Buzzer on an Arduino
Once the passive buzzer is connected, upload this code the Arduino:
int buzzerPin = 8;
void setup() {
pinMode(buzzerPin, OUTPUT);
tone(buzzerPin, 1000, 2000);
}
void loop() {
tone(buzzerPin, 440); // A4
delay(1000);
tone(buzzerPin, 494); // B4
delay(1000);
tone(buzzerPin, 523); // C4
delay(1000);
tone(buzzerPin, 587); // D4
delay(1000);
tone(buzzerPin, 659); // E4
delay(1000);
tone(buzzerPin, 698); // F4
delay(1000);
tone(buzzerPin, 784); // G4
delay(1000);
noTone(buzzerPin);
delay(1000);
}
Explanation of the Code
First we declare a variable for the buzzerPin
and set it equal to Arduino pin 8. Then we set buzzerPin
as an OUTPUT in the setup()
section.
If you want a sound to play when your sketch starts up for the first time, you can put the tone()
function in the setup()
section. Since the setup()
section is executed only once when the sketch starts, the buzzer will produce a single tone at start up. In this sketch, we will have a 1,000 hertz tone play for 2,000 milliseconds at start up.
In the loop()
section we cycle through a scale of seven musical notes, A, B, C, D, E, F and G. The frequencies of each musical note in hertz can be found online. There are seven notes, so we need seven tone()
functions.
Each tone()
function has the first parameter set to output at the buzzerPin
. The second parameter is the frequency in hertz. The A4 note is 440 hertz, so 440
is used as the second parameter in the tone()
function for the A4 musical note. For note B4, the second parameter is 494
, corresponding to 494 hertz. C4 is 523 hertz, and so on all the way up to G4.
The duration parameter doesn’t work very well when the tone()
function is used in the loop()
section. So to set the tone duration in the loop, use a delay()
function after each tone()
function. In this sketch, there is a delay()
function that specifies a 1,000 millisecond (one second) delay after each tone()
function.
At the end of the loop, the noTone()
function and a delay of 1,000 milliseconds is used to add a one second period of silence before the loop repeats.
After you connect the buzzer and upload the code, you should hear the buzzer cycling through the 7 notes in a loop.
Feel free to leave a comment below if you have any questions…