Buzzers are often used in DIY projects to create simple sounds for alarms and alerts. In this tutorial, we will learn how to connect passive and active buzzers to a Raspberry Pi, and how to program them with Python
We will build an example project with an active buzzer that is activated when the reading from a DHT22 humidity and temperature sensor goes above a certain temperature. Then we will build a project with a passive buzzer to play a musical tune.
Active Buzzers vs Passive Buzzers
An active buzzer uses an internal oscillator to generate a tone. This means you only need DC voltage to create a stable sound.
On the other hand, passive buzzers don’t have internal oscillators. You need a pulsating AC signal to generate a stable tone.
Passive buzzers are best when your project requires precise tone and volume control. Active buzzers are best for simple sound generation for alerts and alarms – devices that only produce noise, not music.Â
How to Setup an Active Buzzer on the Raspberry Pi
For our first example, we will use an active buzzer as a sound alert when the reading from a DHT22 sensor goes above a certain temperature.
These are the parts we will need:
Build the circuit following the wiring diagram below:
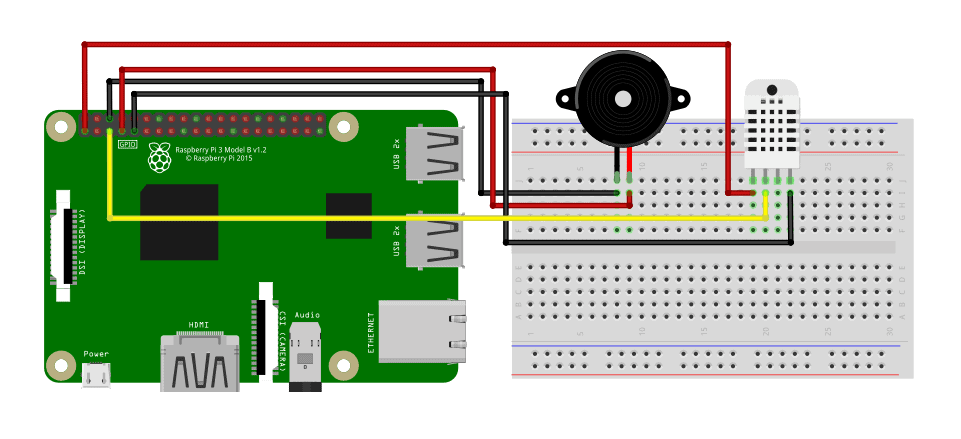
Programming an Active Buzzer
We only need two Python libraries for this project. We will use the Adafruit DHT Python library to interface our DHT22 sensor to the Raspberry Pi, and the RPi.GPIO library to trigger the buzzer.
To install the Adafruit DHT library, enter this command in the terminal:
sudo pip3 install Adafruit_DHT
Code
Copy the code below to your code editor.
import Adafruit_DHT as AdaDHT
import RPi.GPIO as GPIO
DHTSensor = AdaDHT.DHT22
DHTPin = 3
BuzzerPin = 4
GPIO.setwarnings(False)
GPIO.setmode(GPIO.BCM)
GPIO.setup(BuzzerPin, GPIO.OUT, initial=GPIO.LOW)
while True:
humidity, temperature = AdaDHT.read_retry(DHTSensor, DHTPin)
if humidity is not None and temperature is not None:
print("Temperature={0:0.1f}*C Humidity={1:0.1f}%".format(temperature,humidity))
if(temperature>32):
GPIO.output(DHTPin, GPIO.HIGH)
else:
GPIO.output(DHTPin, GPIO.LOW)
else:
print("Failed to retrieve data from sensor")
On line 4 we indicate the DHT sensor we will be using. In this case it’s the DHT22. If you’re using a DHT11, change AdaDHT.DHT22
to AdaDHT.DHT11
. Next, we set the pin numbers for both the sensor and buzzer. Finally, we set the buzzer pin as an output with an initial LOW state.
The main loop reads the temperature and humidity value from the sensor, and if the readings are valid, prints them on the terminal. If the temperature value exceeds 32C, the Raspberry Pi will send a HIGH signal to trigger the active buzzer.
Using a Passive Buzzer
For the next example, we are going use a passive buzzer to play a tune. These are the things we need:
Gather all the items and build the circuit as shown in this wiring diagram:
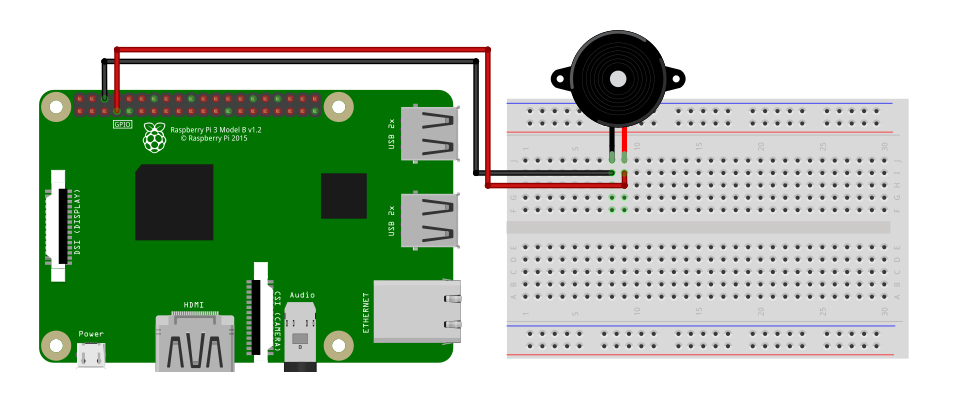
Code
Copy the code below to your code editor.
import RPi.GPIO as GPIO
import time
BuzzerPin = 4
GPIO.setmode(GPIO.BCM)
GPIO.setup(BuzzerPin, GPIO.OUT)
GPIO.setwarnings(False)
global Buzz
Buzz = GPIO.PWM(BuzzerPin, 440)
Buzz.start(50)
B0=31
C1=33
CS1=35
D1=37
DS1=39
E1=41
F1=44
FS1=46
G1=49
GS1=52
A1=55
AS1=58
B1=62
C2=65
CS2=69
D2=73
DS2=78
E2=82
F2=87
FS2=93
G2=98
GS2=104
A2=110
AS2=117
B2=123
C3=131
CS3=139
D3=147
DS3=156
E3=165
F3=175
FS3=185
G3=196
GS3=208
A3=220
AS3=233
B3=247
C4=262
CS4=277
D4=294
DS4=311
E4=330
F4=349
FS4=370
G4=392
GS4=415
A4=440
AS4=466
B4=494
C5=523
CS5=554
D5=587
DS5=622
E5=659
F5=698
FS5=740
G5=784
GS5=831
A5=880
AS5=932
B5=988
C6=1047
CS6=1109
D6=1175
DS6=1245
E6=1319
F6=1397
FS6=1480
G6=1568
GS6=1661
A6=1760
AS6=1865
B6=1976
C7=2093
CS7=2217
D7=2349
DS7=2489
E7=2637
F7=2794
FS7=2960
G7=3136
GS7=3322
A7=3520
AS7=3729
B7=3951
C8=4186
CS8=4435
D8=4699
DS8=4978
song = [
G4,
E4, F4, G4, G4, G4,
A4, B4, C5, C5, C5,
E4, F4, G4, G4, G4,
A4, G4, F4, F4,
E4, G4, C4, E4,
D4, F4, B3,
C4
]
beat = [
8,
8, 8, 4, 4, 4,
8, 8, 4, 4, 4,
8, 8, 4, 4, 4,
8, 8, 4, 2,
4, 4, 4, 4,
4, 2, 4,
1
]
while True:
for i in range(1, len(song)):
Buzz.ChangeFrequency(song[i])
time.sleep(beat[i]*0.13)
We will use two Python libraries as well with this example project. We use the RPi.GPIO library to trigger the buzzer, and the time library to space out the melody’s notes.
For the initial setup, we will define the buzzer pin and the pin numbering system to BCM. We will initialize the PWM as the variable buzz, then set the duty ratio to 50%.
Next, we define all the possible notes we can use to compose a tune by their frequency. The song I used for this example is “Santa Claus is Coming to Town.”
To build the tune, we indicate the notes of the song paired with the beats. These beats are the spacings between notes. In the main loop, every note is sent to the passive buzzer using PWM. Then, the Raspberry Pi will rest in silence according to the respective beat value. You can set the tempo with a constant multiplier.
Hope this has helped you understand how to use active and passive buzzers in your own Raspberry Pi projects! Be sure to leave a comment below if you have questions about anything.
If temperature > 32, shouldn’t you be setting BuzzerPIN rather than DHTpin?